《Core JavaServer Faces 3rd Edition》中第四章的代码:
LocaleChanger.java
package com.corejsf; import java.io.Serializable; import java.util.Locale; import javax.faces.bean.ManagedBean; import javax.faces.bean.SessionScoped; import javax.faces.context.FacesContext; @ManagedBean @SessionScoped public class LocaleChanger implements Serializable { public String germanAction() { FacesContext context = FacesContext.getCurrentInstance(); context.getViewRoot().setLocale(Locale.GERMAN); return null; } public String englishAction() { FacesContext context = FacesContext.getCurrentInstance(); context.getViewRoot().setLocale(Locale.ENGLISH); return null; } public String chineseAction() { FacesContext context = FacesContext.getCurrentInstance(); context.getViewRoot().setLocale(Locale.CHINESE); return null; } }
UserBean.java
package com.corejsf; import java.io.Serializable; import javax.faces.bean.ManagedBean; import javax.faces.bean.SessionScoped; @ManagedBean(name="user") @SessionScoped public class UserBean implements Serializable { private String name; private String password; private String aboutYourself; public String getName() { return name; } public void setName(String newValue) { name = newValue; } public String getPassword() { return password; } public void setPassword(String newValue) { password = newValue; } public String getAboutYourself() { return aboutYourself; } public void setAboutYourself(String newValue) { aboutYourself = newValue; } }
index.xhtml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core"> <h:head> <title>#{msgs.indexWindowTitle}</title> </h:head> <h:body> <h:form> <h:commandLink action="#{localeChanger.germanAction}"> <h:graphicImage library="images" name="de_flag.gif" style="border: 0px; margin-right: 1em;" /> </h:commandLink> <h:commandLink action="#{localeChanger.englishAction}"> <h:graphicImage library="images" name="en_flag.gif" style="border: 0px; margin-right: 1em;" /> </h:commandLink> <h:commandLink action="#{localeChanger.chineseAction}"> <h:graphicImage library="images" name="cn_flag.gif" style="border: 0px; margin-right: 1em;" /> </h:commandLink> <p> <h:outputText value="#{msgs.indexPageTitle}" style="font-style: italic; font-size: 1.3em" /> </p> <h:panelGrid columns="2"> #{msgs.namePrompt} <h:inputText value="#{user.name}" /> #{msgs.passwordPrompt} <h:inputSecret value="#{user.password}" /> #{msgs.tellUsPrompt} <h:inputTextarea value="#{user.aboutYourself}" rows="5" cols="35" /> </h:panelGrid> <h:commandButton value="#{msgs.submitPrompt}" action="success" /> </h:form> </h:body> </html>
thankYou.xhtml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html"> <h:head> <title>#{msgs.thankYouWindowTitle}</title> </h:head> <h:body> <h:outputText value="#{msgs.namePrompt}" style="font-style: italic"/> #{user.name} <br/> <h:outputText value="#{msgs.aboutYourselfPrompt}" style="font-style: italic"/> <br/> <pre>#{user.aboutYourself}</pre> </h:body> </html>
faces-config.xml
<?xml version="1.0"?> <faces-config xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-facesconfig_2_0.xsd" version="2.0"> <navigation-rule> <navigation-case> <from-outcome>success</from-outcome> <to-view-id>/thankYou.xhtml</to-view-id> </navigation-case> </navigation-rule> <application> <locale-config> <default-locale>en</default-locale> <supported-locale>zh</supported-locale> <supported-locale>de</supported-locale> </locale-config> <resource-bundle> <base-name>com.corejsf.messages</base-name> <var>msgs</var> </resource-bundle> </application> </faces-config>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <servlet> <servlet-name>Faces Servlet</servlet-name> <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>Faces Servlet</servlet-name> <url-pattern>/faces/*</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>faces/index.xhtml</welcome-file> </welcome-file-list> <context-param> <param-name>javax.faces.PROJECT_STAGE</param-name> <param-value>Development</param-value> </context-param> </web-app>
运行图片:
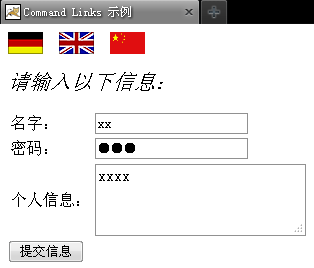